Mit PowerShell und Microsoft Graph können berechtigte Personen Planner Tasks erstellen. In der Vergangenheit konnte eine Beschreibung im Planner Task im Textformat erstellt werden. Die Planner API für plannerTaskDetails inkludiert seit 2022 ein Notes-Property für eine Beschreibung im HTML-Format.
- Property Description > unterstützt nur Plaintext.
- Property Notes > unterstützt HTML-Format.
Wie es Microsoft in der Dokumentation vermerkt, beide Properties sind miteinander verknüpft und dürfen nicht zusammen aktualisiert werden.
notes
Rich text description of the task. To be used by HTML-aware clients. For backwards compatibility, a plain-text version of the HTML description will be synced to the “description” field. If this field hasn’t previously been set but “description” has been, the existing description is synchronized to “notes” with minimal whitespace-preserving HTML markup. Setting both “description” and “notes” is an error and will result in an exception.
Um die Beschreibung von einem Planner Task mit PowerShell zu aktualisieren sind zwei Schritte erforderlich.
- Erstellen von einem Planner Task
- Aktualisieren der Beschreibung des Tasks
Content
Neuer Planner Task mit Beschreibung in Plaintext
Im ersten Beispiel erstelle ich den Task mit dem Property Description. Die Beschreibung von einem Task ist dadurch in Plaintext.
Import-Module Microsoft.Graph.Authentication
Connect-MgGraph -Scopes Tasks.ReadWrite
# Prepare the body to create a new task
$Body = @"
{
"planId": "xWXTRNFiDEuKXzpfad8EV5gAEFfk",
"bucketId": "DvPqFQBk0E-xeopNovLVGpgAEiJv",
"title": "Task with plain description"
}
"@
# Create a new task
$Url = "https://graph.microsoft.com/v1.0/planner/tasks"
$NewPlannerTask = Invoke-MgGraphRequest -Method POST -Uri $Url -Body $Body -ContentType "application/json"
$NewPlannerTaskID = $NewPlannerTask.id
# Get the task details to get the latest etag value (required to update a Planner task)
$Url = "https://graph.microsoft.com/v1.0/planner/tasks/$NewPlannerTaskID/details"
$PlannerTaskDetails = Invoke-MgGraphRequest -Method GET -Uri $Url -ContentType "application/json"
# Prepare the body to update the task with a description
$MessageBody = @"
<p>This is a <strong>sample description</strong> with <em>HTML</em> content.</p>
<ul>
<li>First item</li>
<li>Second item</li>
<li>Third item</li>
</ul>
<p>For more information, visit <a href='https://blog-en.topedia.com/' Target='_blank'>Topedia Blog</a>.</p>
"@
# Update the task body with the description
$Body = @{
'previewType' = 'noPreview'
'description' = $MessageBody
}
# Update the task with the description (in plain text)
$Url = "https://graph.microsoft.com/v1.0/planner/tasks/$NewPlannerTaskID/details"
$Header = @{ "If-Match" = $PlannerTaskDetails.'@odata.etag' }
Invoke-MgGraphRequest -Method PATCH -Uri $Url -Body ($Body | ConvertTo-Json) -Headers $Header -ContentType "application/json"
Der neue Task wurde erstellt. Die Beschreibung ist ein unformatiertes HTML-Format in Plaintext.
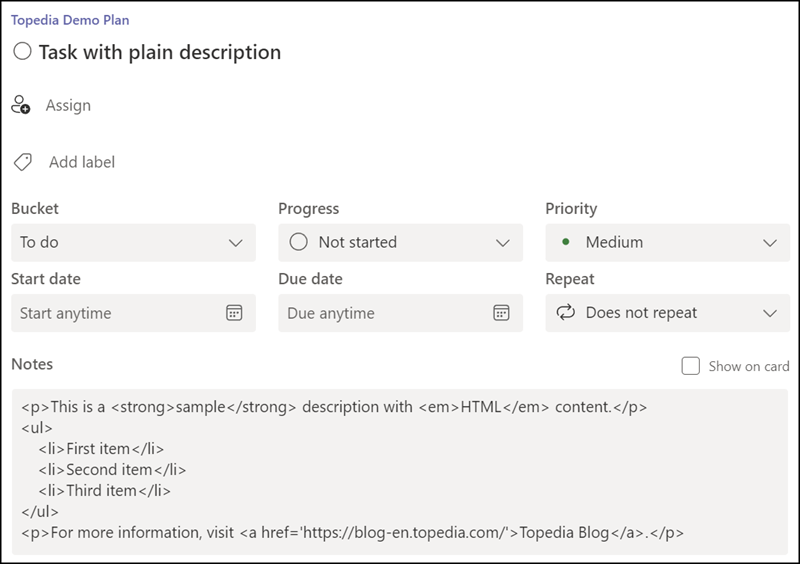
Neuer Planner Task mit Beschreibung im HTML-Format
Derselbe Vorgang für die Erstellung von einem Task mit Beschreibung im HTML-Format.
Wichtig, nutz für das Notes-Property den Beta Endpunkt, sonst wird es die Beschreibung weiterhin in Plaintext erstellen. Der v1 Endpunkt unterstützt bisher kein Notes-Property.
# Prepare the body to create a new task
$Body = @"
{
"planId": "xWXTRNFiDEuKXzpfad8EV5gAEFfk",
"bucketId": "DvPqFQBk0E-xeopNovLVGpgAEiJv",
"title": "Task with HTML description"
}
"@
# Create a new task
$Url = "https://graph.microsoft.com/beta/planner/tasks"
$NewPlannerTask = Invoke-MgGraphRequest -Method POST -Uri $Url -Body $Body -ContentType "application/json"
$NewPlannerTaskID = $NewPlannerTask.id
# Get the task details to get the latest etag value (required to update a Planner task)
$Url = "https://graph.microsoft.com/beta/planner/tasks/$NewPlannerTaskID/details"
$PlannerTaskDetails = Invoke-MgGraphRequest -Method GET -Uri $Url -ContentType "application/json"
# Prepare the body to update the task with a description
$MessageBody = @"
<p>This is a <strong>sample description</strong> with <em>HTML</em> content.</p>
<ul>
<li>First item</li>
<li>Second item</li>
<li>Third item</li>
</ul>
<p>For more information, visit <a href='https://blog-en.topedia.com/'>Topedia Blog</a>.</p>
"@
# Update the task body with the HTML description
$Body = @{
'previewType' = 'noPreview'
'notes' = @{
'contentType' = 'html'
'content' = $MessageBody
}
}
# Update the task with the description (in HTML format)
$Url = "https://graph.microsoft.com/beta/planner/tasks/$NewPlannerTaskID/details"
$Header = @{ "If-Match" = $PlannerTaskDetails.'@odata.etag' }
Invoke-MgGraphRequest -Method PATCH -Uri $Url -Body ($Body | ConvertTo-Json) -Headers $Header -ContentType "application/json"
Disconnect-MgGraph
Der zweite Task wurde erstellt. Die Beschreibung ist ein formatiertes HTML-Format.
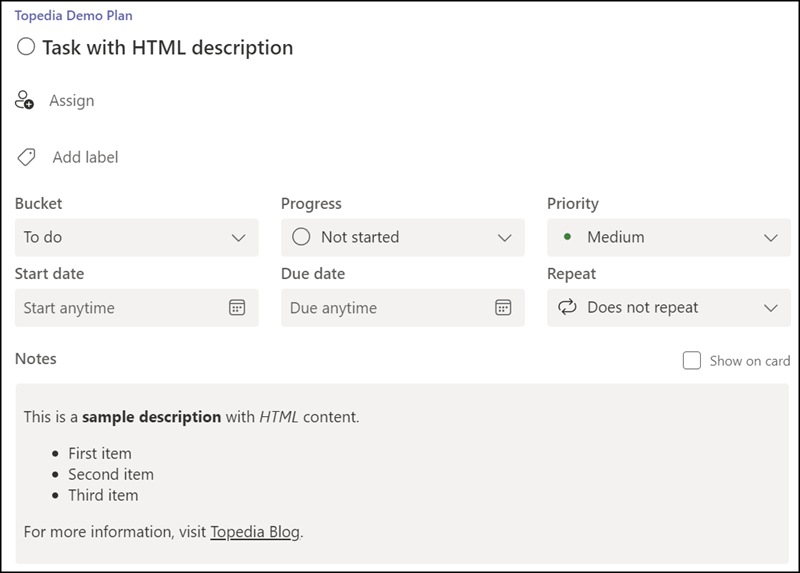
Bonus: Neuer Planner Task aus Microsoft 365 Message im HTML-Format
Die Nachrichten aus dem Microsoft 365 Message Center inkludieren im Body ein HTML-Format. Der ganze Body (inkl. Screenshots) kann im Planner Task erfasst werden.
Beachte, das Notes-Property unterstützt manche HTML-Attribute nicht. Bei Microsoft 365 Messages ist es beispielsweise das Attribut target=”_blank”. In solchen Fällen wird die API einen Fehler zurückgeben.
The request contains invalid HTML for the Notes field. [Invalid or disallowed HTML attribute or attribute value [NotAllowedAttribute]]
In meinem Beispiel für MC949004 entferne ich das Attribut (sofern vorhanden).
Connect-MgGraph -Scopes Tasks.ReadWrite, ServiceMessage.Read.All
# Get the service announcement message for MC949004
$Url = "https://graph.microsoft.com/beta/admin/serviceAnnouncement/messages/MC949004"
$MCResult = Invoke-MgGraphRequest -Method Get -Uri $Url
# Prepare the body to create a new task
$TaskTitle = $MCResult.title + " - " + $MCResult.id
$Body = @"
{
"planId": "xWXTRNFiDEuKXzpfad8EV5gAEFfk",
"bucketId": "DvPqFQBk0E-xeopNovLVGpgAEiJv",
"title": "$TaskTitle"
}
"@
# Create a new task
$Url = "https://graph.microsoft.com/beta/planner/tasks"
$NewPlannerTask = Invoke-MgGraphRequest -Method POST -Uri $Url -Body $Body -ContentType "application/json"
$NewPlannerTaskID = $NewPlannerTask.id
# Get the task details to get the latest etag value (required to update a Planner task)
$Url = "https://graph.microsoft.com/beta/planner/tasks/$NewPlannerTaskID/details"
$PlannerTaskDetails = Invoke-MgGraphRequest -Method GET -Uri $Url -ContentType "application/json"
# Remove an unsupported HTML attribute from the message body
$MessageBody = $MCResult.body.content
$MessageBody = $MessageBody -replace 'target="_blank"', ""
# Prepare the body to update the task with a description
$Body = @{
'previewType' = 'noPreview'
'notes' = @{
'contentType' = 'html'
'content' = $MessageBody
}
}
# Update the task with the description (in HTML format)
$Url = "https://graph.microsoft.com/beta/planner/tasks/$NewPlannerTaskID/details"
$Header = @{ "If-Match" = $PlannerTaskDetails.'@odata.etag' }
Invoke-MgGraphRequest -Method PATCH -Uri $Url -Body ($Body | ConvertTo-Json) -Headers $Header -ContentType "application/json"
Disconnect-MgGraph
Der dritte Task wurde erstellt und die Beschreibung im HTML-Format übernommen.
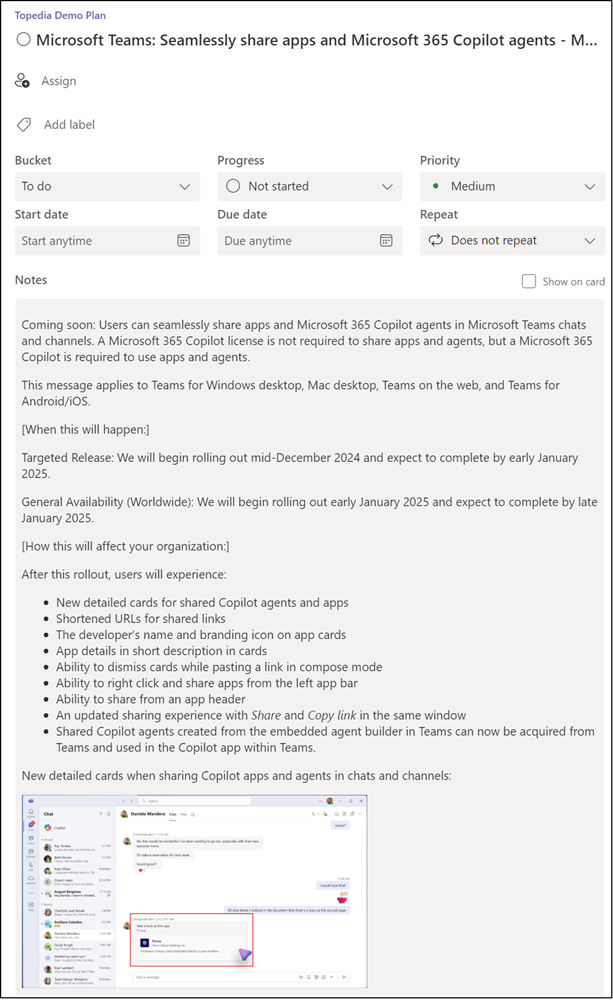